This guide will help you integrate PDF.js Express Viewer into Blazor applications from scratch. You can find an existing project guide here. It will help you clone the Blazor sample repository, walk through the project structure, and show you how to call other WebViewer API.
Get the Blazor sample source code herePrerequisites
Initial setup
Clone the Blazor sample by executing
git clone https://github.com/pdfjs-express/pdfjs-express-viewer-blazor-sample.git
Once cloned, navigate into the
pdfjs-express-viewer-blazor-sample
directory and install all the required dependencies by executingnpm install
npm will also download the PDF.js Express WebViewer and extract it to
/wwwroot/lib
.
You are now ready to run the sample or use more WebViewer APIs.
Sample overview
After initial setup, the pdfjs-express-viewer-blazor-sample
directory should look something like this:
pdfjs-express-viewer-blazor-sample
├───App.razor
├───BlazorWebViewerServer.csproj
├───Program.cs
├───Startup.cs
├───Data
│ └───...
├───Pages
│ ├───Counter.razor
│ ├───Error.razor
│ ├───FetchData.razor
│ ├───Index.razor
│ ├───WebViewer.razor
│ └───_Host.cshtml
│
├───Properties
│ └───launchSettings.json
│
├───Shared
│ ├───MainLayout.razor
│ └───NavMenu.razor
└───wwwroot
| ├───webviewer-demo.pdf
| ├───css
| │ ├───site.css
| | └───...
| ├───js
| │ └─── webviewerScripts.js
| ├───lib
| | └───...
| └───...
└───...
Notable files and directories include:
File/Folder Name | Description |
---|---|
wwwroot | Contains all the static files and PDF.js Express WebViewer library |
Shared | Contains the main layout for all the pages |
Pages | Contains the html and C# code for all the pages |
Startup.cs | Contains the configuration for the ASP.NET server |
_Host.cshtml | Contains the main HTML layout for the application |
In short, webviewerScripts.js
contains the JavaScript code for instantiating and interacting with WebViewer. WebViewer.razor
then calls those functions via JavaScript interop.
Run the sample
To run the sample, navigate to your pdfjs-express-viewer-blazor-sample/
directory and execute
npm start
Then navigate to https://localhost:5001/webviewer
. You should see the application load on your browser.
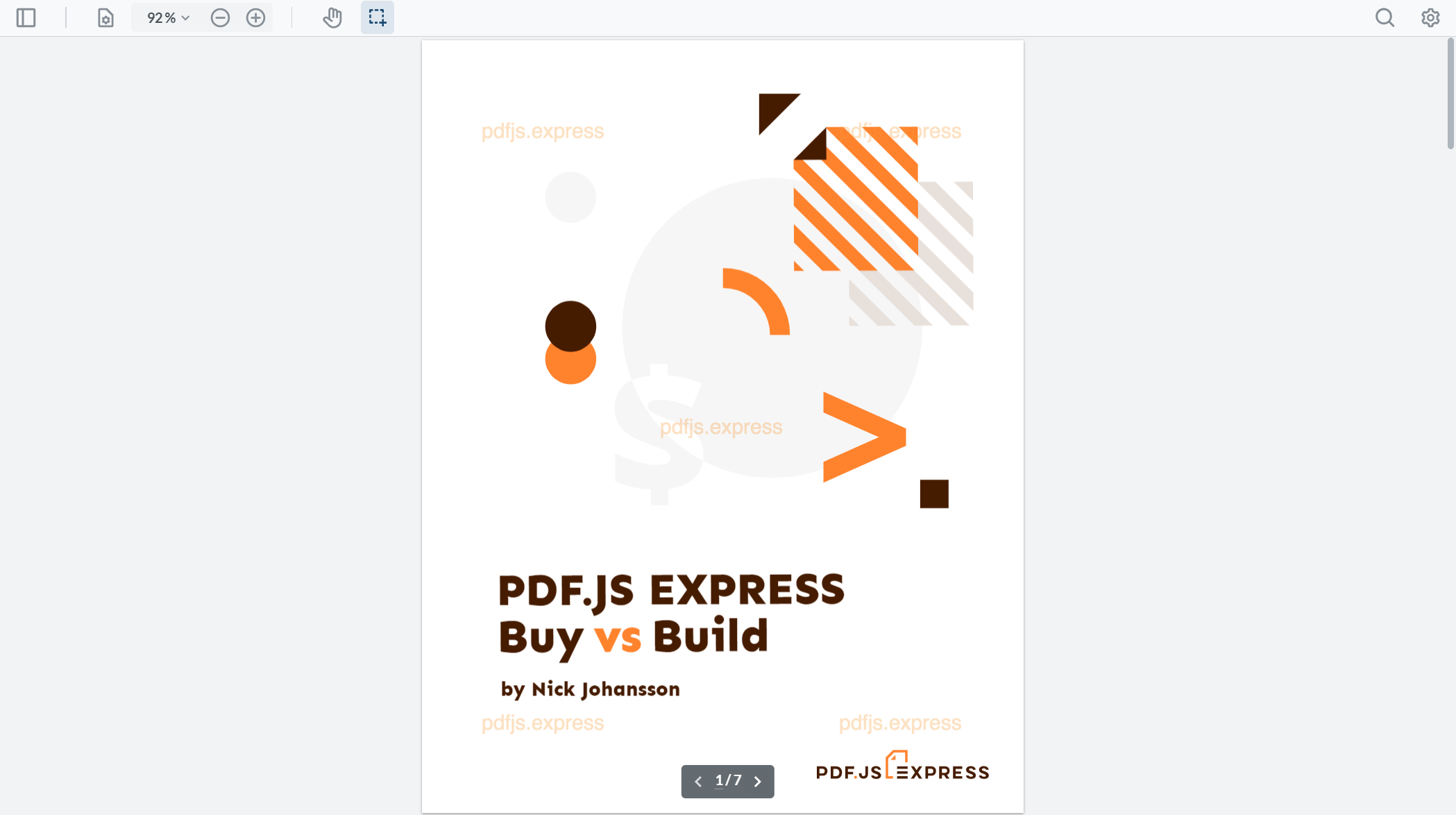
Use more WebViewer APIs
To call more WebViewer API's, navigate to /wwwroot/js/webviewerScripts.js
and add the API calls in the callback of the WebViewer constructor(you may need to make one if it is not provided).
initWebViewer: () => {
const viewerElement = document.getElementById('viewer');
WebViewer({
path: 'WebViewer/lib',
initialDoc: 'https://pdftron.s3.amazonaws.com/downloads/pl/demo-annotated.pdf'
}, viewerElement).then(instance => {
// call apis here
})
}
For example, you can add instance.setTheme('dark')
to change the WebViewer UI theme to dark. If you stop the server, and execute dotnet run
again, then reload the page, you should see the theme change:
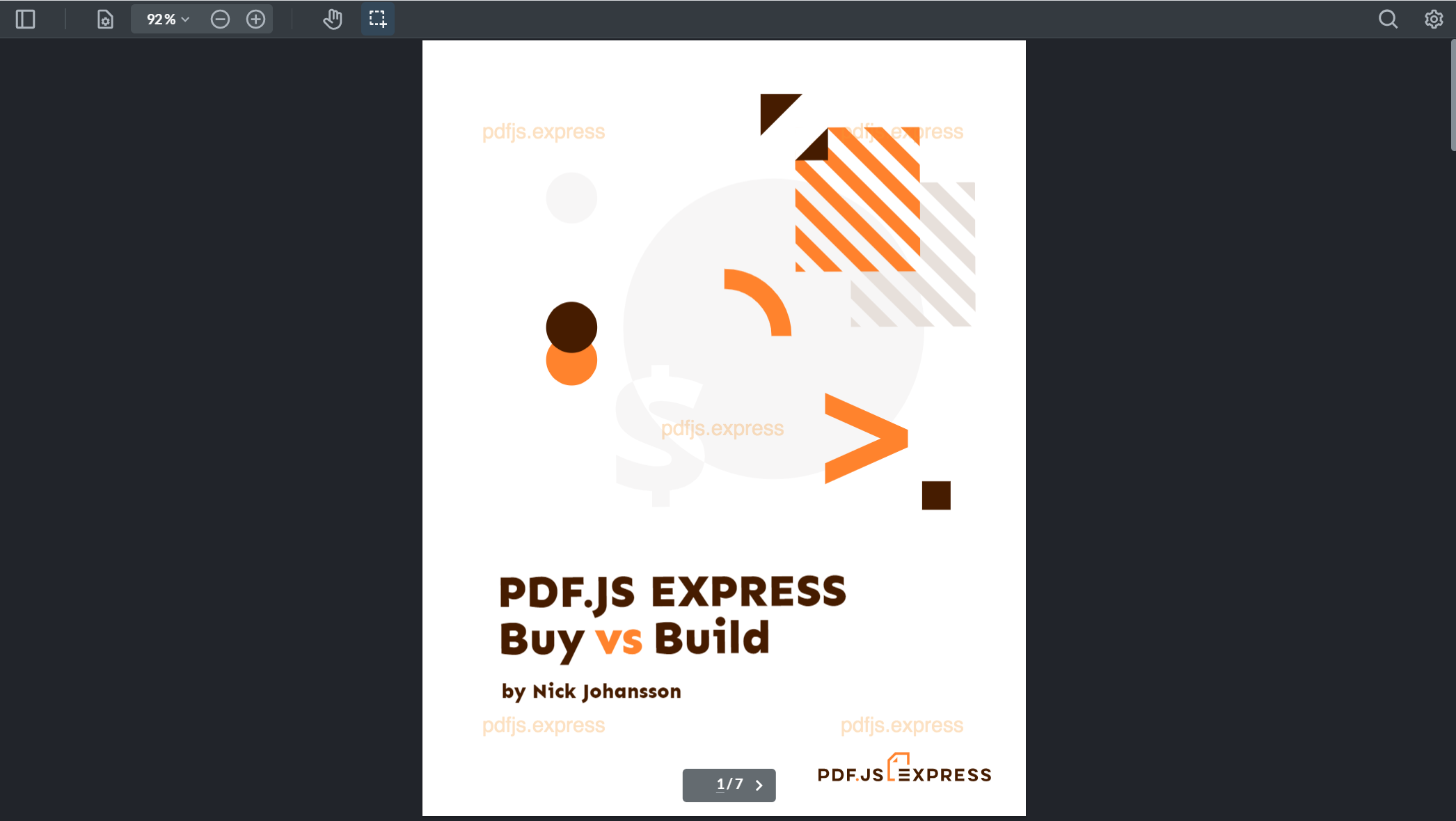
Next step
GuidesSamplesAPI docsTroubleshooting
MIME issues
Enable MIME type mappings for WebViewer to load documents.
Server-side document operations with .NET Core SDK
Handle document operations through the JavaScript interop by passing its base64 data.