This guide will help you integrate PDF.js Express Viewer into Svelte applications on the browser. It will help you clone the Svelte sample repository, walk through the project structure, and show you how to call other WebViewer API.
Get the Svelte sample source code herePrerequisites
Initial setup
Clone the Svelte sample by executing
git clone https://github.com/pdfjs-express/pdfjs-express-viewer-svelte-sample.git
Once cloned, navigate into the
pdfjs-express-viewer-svelte-sample
folder and install all the required dependencies by executingnpm install
npm will also download the PDF.js Express Viewer and extract it to /public/lib
.
You are now ready to run the sample or use more WebViewer APIs.
Sample overview
After initial setup, your pdfjs-express-viewer-svelte-sample
directory should be laid out like this:
pdfjs-express-viewer-svelte-sample
├── LICENSE
├── node_modules
│ └── ...
├── package.json
├── package-lock.json
├── public
│ ├── bundle.css
│ ├── bundle.css.map
│ ├── bundle.js
│ ├── bundle.js.map
│ ├── favicon.png
│ ├── global.css
│ ├── index.html
│ └── lib
├── README.md
├── rollup.config.js
└── src
├── App.svelte
├── main.js
└── WebViewer.svelte
Notable files and directories include:
File/Folder Name | Description |
---|---|
LICENSE | Lists the PDF.js Express copyright and license information. |
App.svelte | Contains the main application code which instantiates a WebViewer and loads a document |
main.js | Adds the application defined in App.svelte to the body element of /public/index.html |
WebViewer.svelte | Contains the definition for the WebViewer component |
bundle.js | JavaScript bundle that is compiled by Svelte |
lib | Contains the extracted PDF.js Express SDK |
Run the sample
To run the sample, navigate to your /pdfjs-express-viewer-svelte-sample
directory and execute
npm run dev
Then navigate to http://localhost:5000
. You should see WebViewer start up and load the sample PDF. Note it will change in real time if you edit any of the source files.
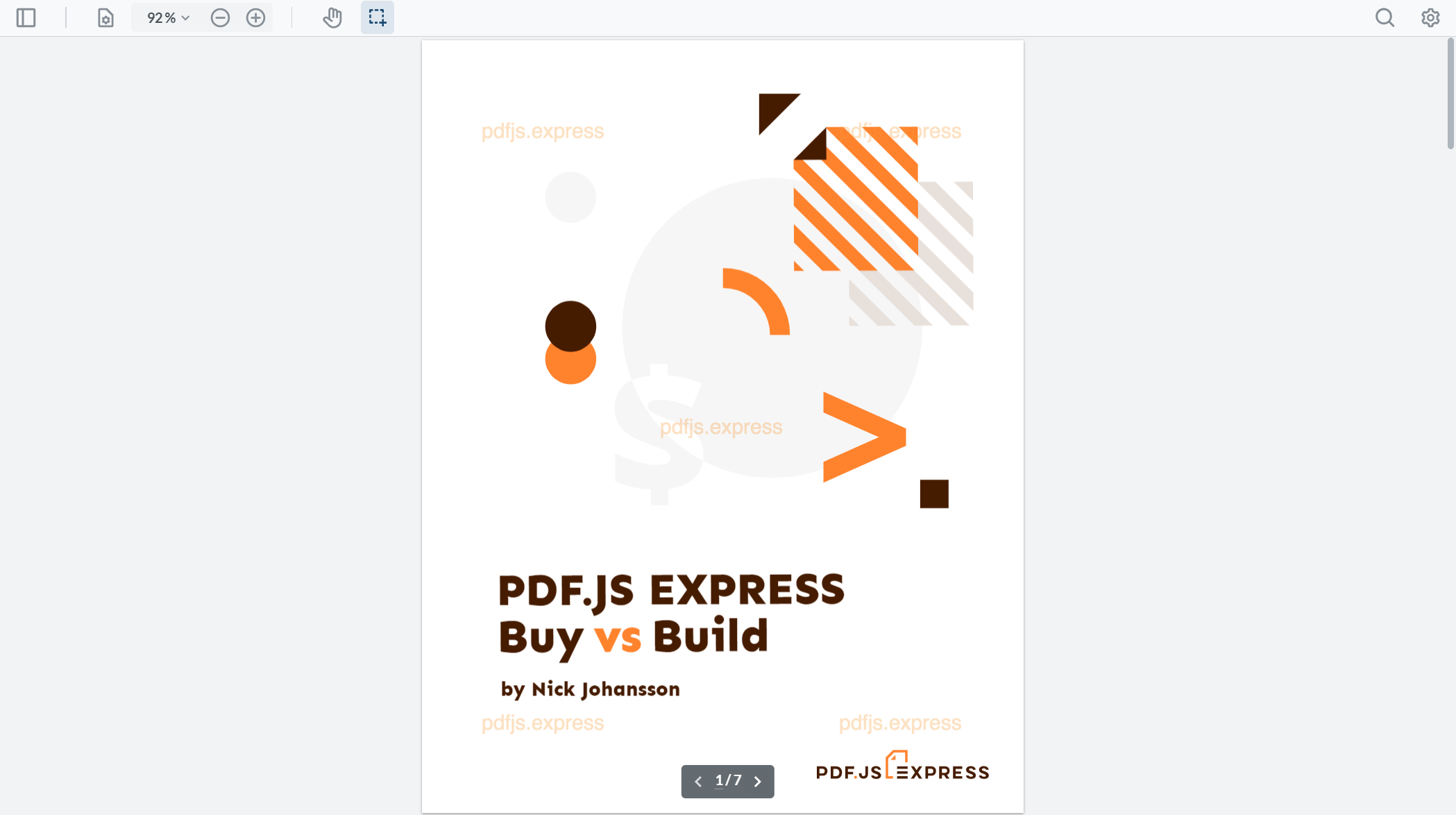
Use more WebViewer APIs
To call more WebViewer API's, navigate to /src/WebViewer.svelte
and add the API calls in the callback of the WebViewer constructor(you may need to make one if it is not provided).
const ele = document.getElementById('viewer');
window.WebViewer({
path: '/lib'
}, ele)
.then(instance => {
// In this callback WebViewer will be initialized, so you can call WebViewer API here.
instance.UI.setTheme('dark');
});
const ele = document.getElementById('viewer');
window.WebViewer({
path: '/lib'
}, ele)
.then(instance => {
// In this callback WebViewer will be initialized, so you can call WebViewer API here.
instance.UI.setTheme('dark');
});
If your Svelte application is already running, you should see the theme change right away. Otherwise execute npm run dev
again, then navigate to http://localhost:5000
and you should see that the theme has changed.
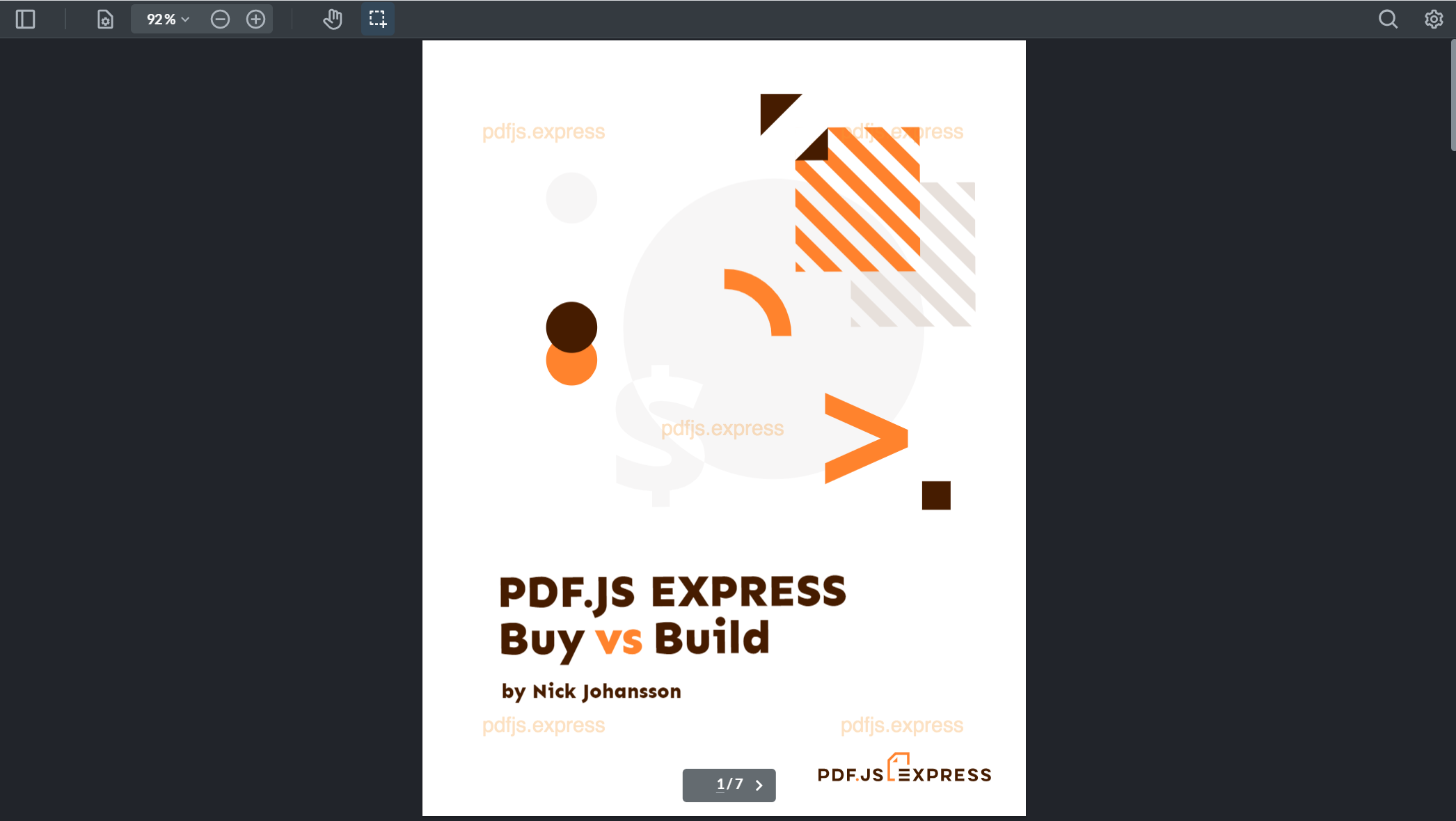